Javascript Coding Standard — Clean Code
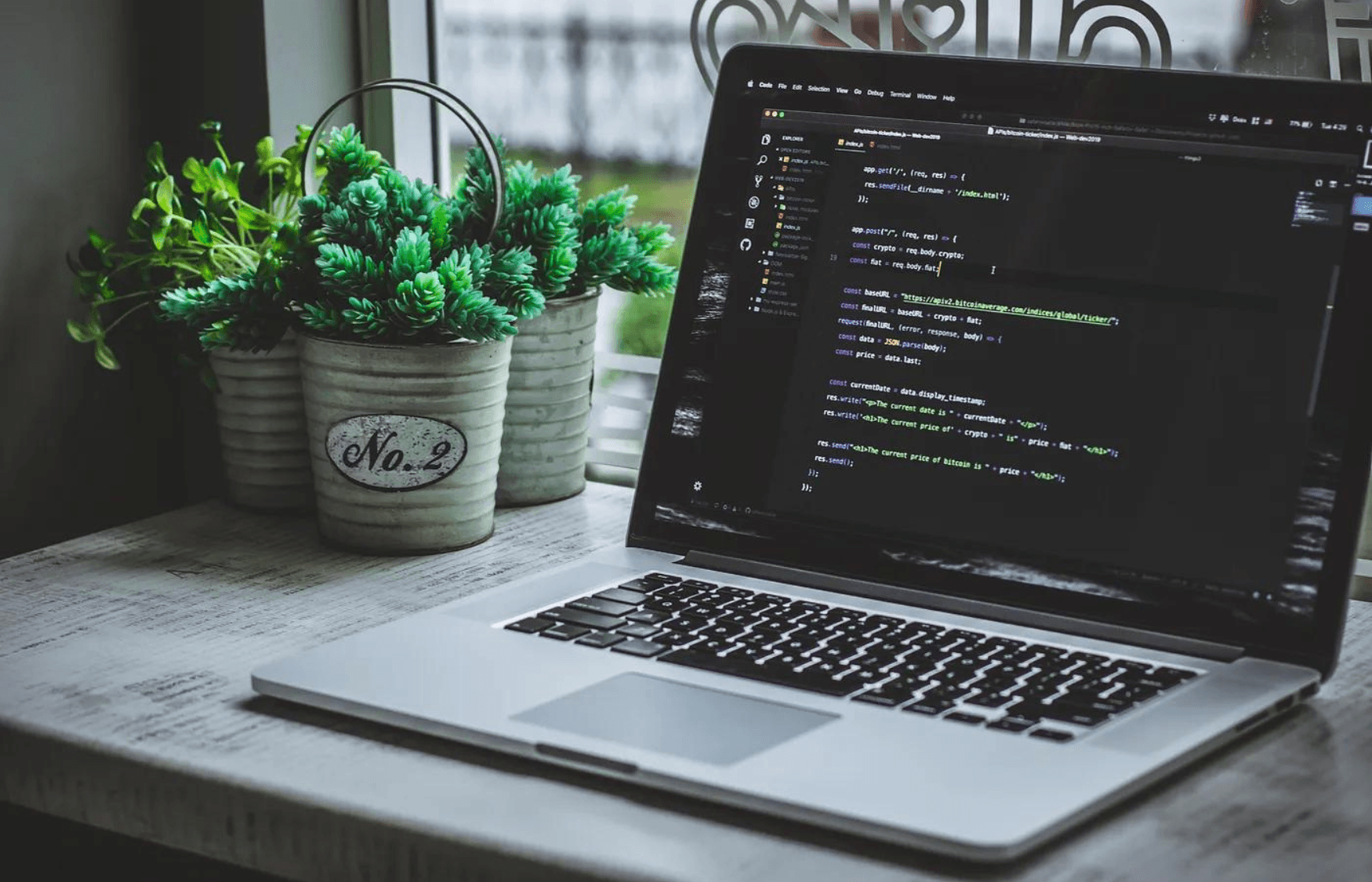
Keep your code readable, changeable, extensible, and maintainable. 😊
The code is clean if it can be understood easily by everyone. Clean Code can be read and enhanced by a developer other than its original author.
The benefits of writing clean code are:
- Easier to start or continue
- Better for team onboarding
- Easier to follow
Here are some of my tips for coding standards.
1. Always use ‘let’ instead of ‘var’
Always use let instead of var because of scoping issues in JavaScript.
1var ex1 = 'Medium';
2let ex2 = 'Medium';
2. Always use ‘const ’ instead of ‘let’
Always use const which stops developers trying to change that shouldn’t and really helps with readability.
1let TAX_RATE = 13;
2const TAX_RATE = 13;
3. Always use a semicolon (;)
As semicolon is optional in Javascript, it is good practice to use a semicolon(;) which helps to keep our code consistent.
1let a = 5;
2if(a > 10){
3 a = a * 2;
4 console.log(a);
5}
4. Always use === when comparing instead of ==
As == allows coercion which might give you unexpected results, always use === which checks a value and type equality.
1let a = '42';
2let b = 42;
3a == b //true
4a === b // false
5. Naming Conventions
Always use camelCase while declaring variables and functions. If a constant variable is at the top of the file use the upper-snake case. Use PascalCase in class.
1const TAX_RATE = 13;
2let myCar = new Car('BMW');
3function randomRange(min, max){
4 return Math.floor(Math.random() * (max - min + 1) + min);
5}
6class MyClass {
7 constructor(name, age){
8 this.name = name;
9 this.age = age;
10 }
11 printName(){
12 console.log(this.name)
13 }
14}
6. Always use template literals when concatenating
Template literals are string literals allowing embedded expressions. You can use multi-line strings and string interpolation features with them.
1const FIRST_NAME = 'John';
2const LAST_NAME = 'Lennon';
3
4const fullName = `${FIRST_NAME} ${LAST_NAME}`; // 'John Lennon'
7. Always use ES6 arrow functions where possible
An arrow function expression is a syntactically compact alternative to a regular function expression, although without its own bindings to this, arguments, super, or new. target keywords.
Arrow functions make our code more concise and simplify function scoping and this keyword.
1// Normal function
2let multiply = function(x, y){
3 return x * y;
4}
5
6// Arrow function
7let multiply = (x, y) => x * y;
8. Always use curly braces in control statements
Always use curly braces in all the control statements such as if-else, for, do, while, etc. which prevents us from silly mistakes. Also, make sure that curly braces start in the same line with space in between.
1let time = 7;
2
3if (time < 10) {
4 greeting = "Good morning";
5} else if (time < 20) {
6 greeting = "Good day";
7} else {
8 greeting = "Good evening";
9}
9. Always try to reduce deep nesting
An if within if can get messy and very hard to read and sometimes you may not be able to get around.
1if(price > 0){
2 if(price > 100){
3 if(!hasDiscount){
4 return addDiscount(0)
5 } else {
6 return addDiscount(10);
7 }
8 } else if ( price > 50){
9 if(!hasDiscount){
10 return addDiscount(5);
11 } else {
12 if(!hasDiscount) {
13 return addDiscount(0);
14 } else {
15 return addDiscount(1);
16 }
17 }
18 } else {
19 error();
20 }
21}
So try to reduce nesting as much as possible which keeps your code readable.
1if(price <= 0){
2 return error;
3}
4if(!hasDiscount){
5 return addDiscount(0);
6}
7if(price > 100){
8 return addDiscount(10);
9}
10if(price > 50){
11 return addDiscount(5)
12}
13return addDiscount(1)
10. Always use Ternary Operator if possible
It is a best practice to use the ternary operator when it makes the code easier to read. If the logic contains many if…else statements, you shouldn’t use the ternary operators.
1function getFee(isMember) {
2 return (isMember ? '$2.00' : '$10.00');
3}
4
5console.log(getFee(true));
6// expected output: "$2.00"
7
8console.log(getFee(false));
9// expected output: "$10.00"
11. Always use default parameters where possible
In JavaScript, function parameters default to undefined. However, it’s often useful to set a different default value. This is where default parameters can help.
1function str(name) {
2 console.log(name);
3}
4str(); // undefined
5
6function str(name = 'Brad') {
7 console.log(name)
8}
9str(); // 'Brad'
12. Always use break and default in switch statements
A switch statement can replace multiple if checks. It gives a more descriptive way to compare a value with multiple variants. Make sure to use break and default from getting ‘fall through’.
1let day;
2switch (new Date().getDay()) {
3 case 0:
4 day = "Sunday";
5 break;
6 case 1:
7 day = "Monday";
8 break;
9 case 2:
10 day = "Tuesday";
11 break;
12 case 3:
13 day = "Wednesday";
14 break;
15 case 4:
16 day = "Thursday";
17 break;
18 case 5:
19 day = "Friday";
20 break;
21 case 6:
22 day = "Saturday";
23 break;
24 default:
25 day = "Unknown Day";
26}
13. Always declare one variable per declaration
It gets messy when you declare more than one variable at a time.
1// let a = 10, b = 20;
2
3let a = 10;
4let b = 20;
14. Try to use named exports instead of the default exports
Using default export can sometimes lead to inconsistency. Give a specific name while importing modules.
1// export default class MyClass{}
2
3export class MyClass{}
15. Do not use wildcard imports
Wildcard imports can sometimes cause name conflicts and ambiguities. Two classes with the same name, but in different packages, will need to be specifically imported, or at least specifically qualified when used.
1// import * as Foo from './foo';
2
3import Foo from './foo';
Conclusion
- Make code readable for people
- Use meaningful names for variables, functions, and methods
- Let one function or method perform only one task
- Be consistent
- Review your code regularly
I hope that these practices or tips will be enough to help you get started with writing clean code. Now, like with everything, the most important thing is to get started. So, practice and try it out. 😉