JavaScript Optimization Techniques for Fast Web Performance
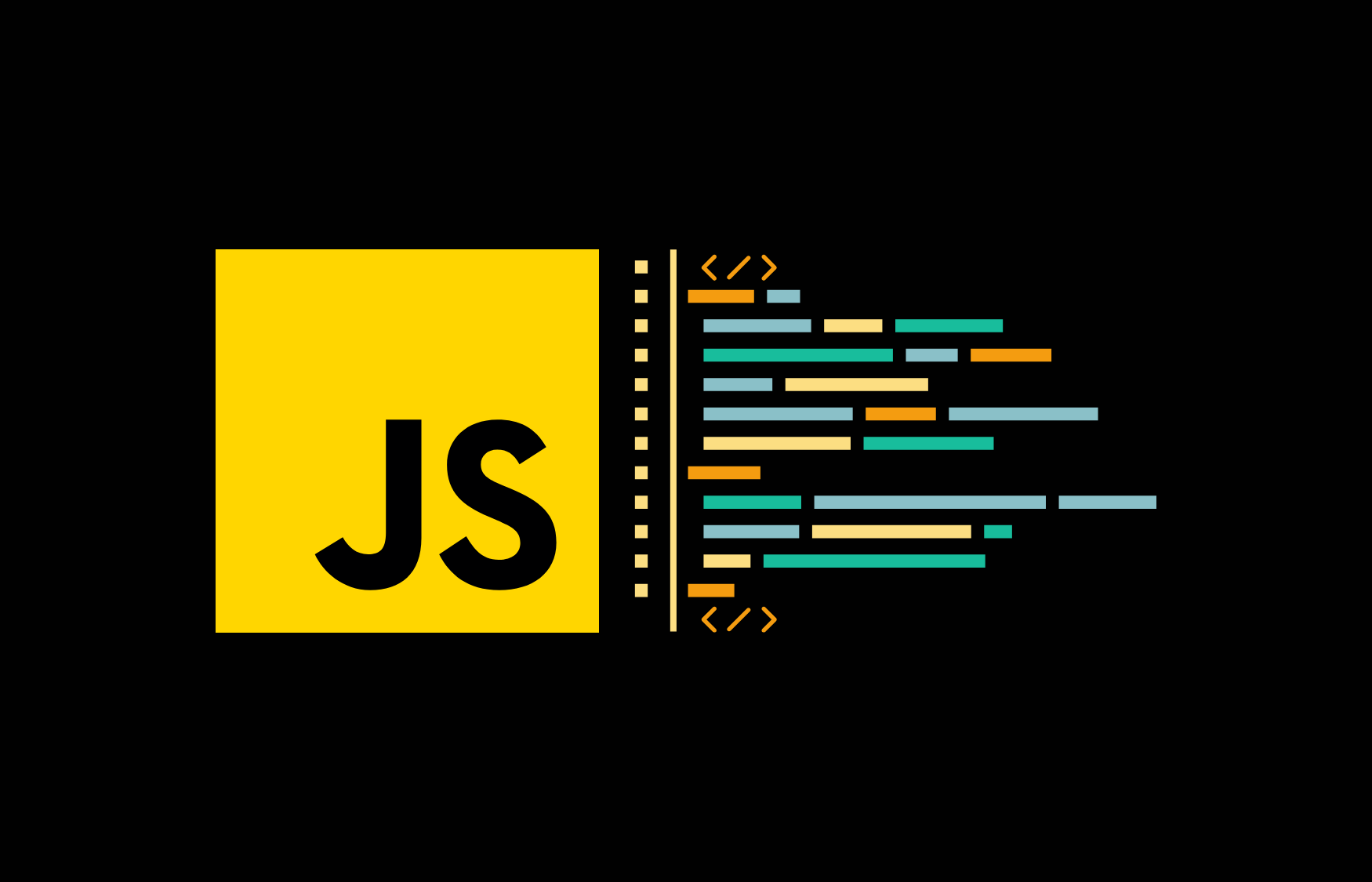
In the dynamic world of web development, performance is not merely an attribute — it’s the cornerstone of user satisfaction. A well-optimized web application ensures a seamless, responsive user experience, fostering user engagement and satisfaction. This article covers foundational and advanced optimization techniques, using simple code examples to illustrate how these techniques can be effectively implemented to build a more efficient and high-performing web application.
Foundational Optimization Techniques
1. Minimize DOM Access
Why: DOM operations are expensive. Excessive DOM manipulation can lead to significant performance bottlenecks, affecting user experience due to layout thrashing and unnecessary reflows.
Strategy: Cache DOM references in variables and batch DOM updates. By minimizing direct DOM manipulation, you can significantly reduce costly document reflows and repaints.
Example:
1// Inefficient DOM access
2for (let i = 0; i < 1000; i++) {
3 document.getElementById('myElement').innerText = i;
4}
5
6// Optimized DOM access
7const myElement = document.getElementById('myElement');
8for (let i = 0; i < 1000; i++) {
9 myElement.innerText = i;
10}
This approach minimizes the number of times the DOM is accessed and updated, leading to improved performance.
2. Use Event Delegation
Why: Adding event listeners to each element separately can cause memory bloat and decrease performance, especially when dealing with a large number of elements.
Strategy: Attach a single event listener to a parent element and manage interactions using the event’s target. This technique, known as event delegation, significantly reduces the total number of event listeners and conserves memory.
Example:
1// Without event delegation
2document.querySelectorAll('.button').forEach(button => {
3 button.addEventListener('click', handleButtonClick);
4});
5
6// With event delegation
7document.getElementById('buttonContainer').addEventListener('click', (event) => {
8 if (event.target.classList.contains('button')) {
9 handleButtonClick(event);
10 }
11});
3. Avoid Global Variables
Why: Global variables can lead to naming conflicts, are slower to access due to scope chain traversal, and can make your codebase less maintainable and more error-prone.
Strategy: Use local variables wherever possible and encapsulate your code within functions or modules. This approach helps maintain a clean global namespace and reduces the risk of variable conflicts.
Example:
1// Global variable - Not recommended
2let counter = 0;
3
4// Encapsulated code block
5(() => {
6 let counter = 0;
7 // Rest of your code
8})();
4. Refactor and Remove Unused Code
Why: Dead code adds unnecessary bytes to your JavaScript files, increasing load times and potentially leading to confusion and maintenance difficulties.
Strategy: Regularly review and clean up your codebase. Utilize tools like ESLint for code quality checks and tree-shaking utilities in module bundlers to eliminate dead code.
Tools like ESLint and Webpack can assist in identifying and removing unused code during the build process.
5. Cache Variables
Why: Repeatedly accessing DOM properties or computing complex values can be highly inefficient and slow down your application.
Strategy: Cache results of expensive operations or frequently accessed properties in variables to avoid redundant calculations and DOM queries.
Example:
1// Accessing DOM property multiple times
2for (let i = 0; i < 1000; i++) {
3 if (document.getElementById('myElement').clientWidth > 100) {
4 // Perform operation
5 }
6}
7
8// Caching DOM property
9const myElementWidth = document.getElementById('myElement').clientWidth;
10for (let i = 0; i < 1000; i++) {
11 if (myElementWidth > 100) {
12 // Perform operation
13 }
14}
6. Use For Loops Instead of Foreach
Why: Traditional for
loops are generally faster than forEach
because forEach
involves creating a new function scope for each iteration.
Strategy: Use for
loops when dealing with performance-sensitive parts of your code or when iterating over large datasets.
Example:
1const myArray = [1, 2, 3, 4, 5];
2
3// Using forEach
4myArray.forEach(item => {
5 console.log(item);
6});
7
8// Using for loop
9for (let i = 0; i < myArray.length; i++) {
10 console.log(myArray[i]);
11}
7. Use the Latest Version of JavaScript
Why: Modern JavaScript versions introduce new features and syntactic improvements that can enhance code efficiency and readability.
Strategy: Adopt ES6+ features where appropriate, such as arrow functions, template literals, and destructuring. Use tools like Babel to transpile your code for compatibility with older browsers.
Example:
1// Old syntax (ES5)
2function add(a, b) {
3 return a + b;
4}
5
6// Modern syntax (ES6+)
7const add = (a, b) => a + b;
8. Optimize Conditional Statements
Why: Efficiently structured conditional statements can lead to faster decision-making in your code.
Strategy: Arrange conditions logically, placing the most likely to be true, or the least costly in terms of performance, at the beginning. Consider using switch
statements or lookup objects for scenarios involving numerous conditions.
Example:
1const fruitColor = {
2 apple: 'red',
3 banana: 'yellow',
4 grape: 'purple',
5};
6
7function getFruitColor(fruit) {
8 return fruitColor[fruit] || 'unknown';
9}
9. Minimize Network Requests
Why: Excessive or large network requests can drastically slow down your application, leading to longer load times and a poor user experience.
Strategy: Utilize build tools like Webpack, Rollup, or Parcel to bundle and minify your JavaScript files. Serve your files with GZIP or Brotli compression to minimize the data transferred.
10. Optimize Animation and Effects
Why: Smooth animations and visual effects are crucial for a polished user experience. Poorly optimized animations can lead to choppy and unappealing visual effects.
Strategy: Utilize requestAnimationFrame
for creating smooth and efficient animations. For simpler animations and transitions, consider using CSS transitions or keyframe animations.
Example:
1function animate() {
2 // Animation logic here
3 requestAnimationFrame(animate);
4}
5
6requestAnimationFrame(animate);
Advanced Optimization Techniques
1. Debouncing:
Purpose: Debouncing is a technique used to ensure that a function does not fire too quickly and too often. It’s particularly useful for events that trigger many times within a short duration, such as window resizing, scrolling, or keypress events in search inputs.
How It Works: Debouncing enforces a delay before executing the function after the last event. If a new event is triggered during the delay, the timer resets. This way, the function is not called until the events have stopped for a specific amount of time.
Implementation:
1const debounce = (func, delay) => {
2 let inDebounce;
3 return function() {
4 const context = this;
5 const args = arguments;
6 clearTimeout(inDebounce);
7 inDebounce = setTimeout(() => func.apply(context, args), delay);
8 };
9};
10
11// Example usage: Debouncing a resize event handler
12const handleResize = debounce(() => {
13 // Handle resize event
14}, 300);
15window.addEventListener('resize', handleResize);
Throttling:
Purpose: Throttling is similar to debouncing but with a slightly different approach. While debouncing delays the function execution until after the events have stopped for a specified duration, throttling ensures that the function is executed at most once every specified interval, regardless of how many times the event fires.
How It Works: Throttling uses a flag or a timestamp to allow function execution at controlled intervals. If the function is called before the interval has passed, it will be ignored or postponed until the interval completes.
Implementation:
1const throttle = (func, limit) => {
2 let inThrottle;
3 return function() {
4 const args = arguments;
5 const context = this;
6 if (!inThrottle) {
7 func.apply(context, args);
8 inThrottle = true;
9 setTimeout(() => (inThrottle = false), limit);
10 }
11 };
12};
13
14// Example usage: Throttling a scroll event handler
15const handleScroll = throttle(() => {
16 // Handle scroll event
17}, 300);
18window.addEventListener('scroll', handleScroll);
Memoization:
Purpose: Memoization is an optimization technique used to increase the efficiency of computationally intensive functions by storing the results of function calls and returning the cached result when the same inputs occur again.
How It Works: Memoization involves creating a cache (usually an object or a map) to store the results of function calls with specific arguments. If the function is called again with the same arguments, the cached result is returned, avoiding the need to recompute.
Implementation:
1const memoize = (func) => {
2 const cache = {};
3 return function(...args) {
4 const key = JSON.stringify(args);
5 if (!cache[key]) {
6 cache[key] = func.apply(this, args);
7 }
8 return cache[key];
9 };
10};
11
12// Example usage: Memoizing an expensive computation function
13const expensiveFunction = memoize((num) => {
14 // Expensive computation here
15 return num * num;
16});
Lazy Loading:
Purpose: Lazy loading is a design pattern used to defer the initialization or loading of resources until they are needed. This technique can significantly improve performance, especially in applications that require loading large amounts of data or media.
How It Works: Lazy loading delays the loading of non-critical resources at page load time and loads them only when they are needed. This reduces the initial load time and saves bandwidth.
Implementation:
1// Using Intersection Observer API for lazy loading images
2const images = document.querySelectorAll("[data-src]");
3const config = {
4 rootMargin: '0px 0px 50px 0px',
5 threshold: 0
6};
7
8let observer = new IntersectionObserver((entries, self) => {
9 entries.forEach(entry => {
10 if (entry.isIntersecting) {
11 const src = entry.target.getAttribute('data-src');
12 if (src) {
13 entry.target.src = src;
14 self.unobserve(entry.target);
15 }
16 }
17 });
18}, config);
19
20images.forEach(image => {
21 observer.observe(image);
22});
23
Conclusion
By integrating these foundational and advanced techniques into your development practices, you enhance the performance and responsiveness of your JavaScript-driven applications and ensure a seamless, efficient, and enjoyable experience for your users.
Remember, performance optimization is an ongoing process. Regularly monitoring, profiling, and refining your code is crucial to ensuring your application remains efficient, responsive, and ahead of the curve.
Happy coding!