Understanding .bind(), .call(), and .apply() Methods
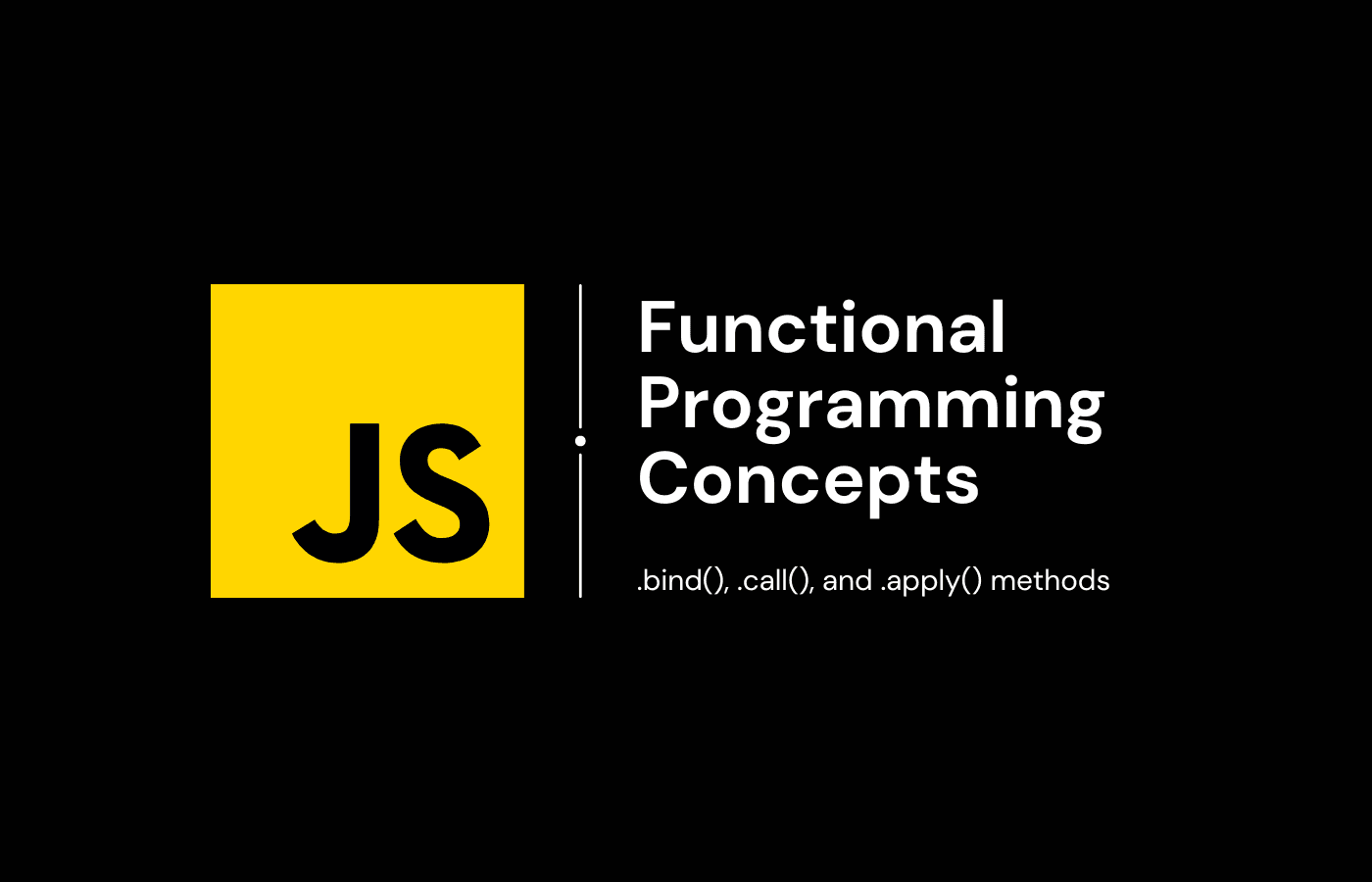
JavaScript, with its versatile ecosystem, offers multiple ways to manipulate and handle functions. Among them, JavaScript provides three powerful methods for changing how functions work: .bind()
, .call()
, and .apply()
. While seemingly straightforward, these methods open up a vast array of possibilities for developers when working with functions. Let’s dive deep into these methods, explore their use cases, and understand how they fit into the function prototype.
The Basics of Context in JavaScript
Before diving into the specific methods, it's essential to grasp the concept of this
in JavaScript. The this
keyword refers to the object that is executing the current function. The value of this
is determined by how a function is invoked, and this is where .bind()
, .call()
, and .apply()
come into play. They allow developers to explicitly set the this
value, enabling more flexible and dynamic code execution.
The .bind()
Method
The .bind()
method creates a new function that, when called, has its this
keyword set to the provided value, with a given sequence of arguments preceding any provided when the new function is called.
Syntax
1const boundFunction = originalFunction.bind(thisArg[, arg1[, arg2[, ...]]]);
thisArg
: The value to be passed as thethis
parameter to the target function when the bound function is called.arg1, arg2, ...
: Arguments to prepend to arguments provided to the bound function when invoking the original function.
Use Case
Imagine you're working with an object that has a method requiring a callback function. You want the callback to have access to the object through this
. .bind()
is perfect for this:
1const person = {
2 name: 'Rohan Shakya',
3 greet: function() {
4 console.log(`Hello, ${this.name}`);
5 }
6};
7
8setTimeout(person.greet.bind(person), 1000);
In this example, .bind()
ensures that person.greet
maintains person
as its context, even when executed asynchronously via setTimeout
.
The .call()
Method
The .call()
method calls a function with a given this
value and arguments provided individually.
Syntax
1function.call(thisArg[, arg1[, arg2[, ...]]]);
2
thisArg
: The value to use asthis
for the function call.arg1, arg2, ...
: Arguments for the function.
Use Case
.call()
is incredibly useful for method borrowing, where you want to use a method from one object on another:
1function introduce(label) {
2 console.log(`${label}: My name is ${this.name}`);
3}
4
5const person = {
6 name: 'Rohan Shakya'
7};
8
9introduce.call(person, 'Introduction');
This snippet shows how .call()
can invoke the introduce
function, explicitly setting its this
to person
and passing additional arguments as needed.
The .apply()
Method
The .apply()
method is similar to .call()
, but instead of passing arguments individually, it takes an array of arguments.
Syntax
1function.apply(thisArg, [argsArray]);
2
thisArg
: The value to use asthis
for the function call.argsArray
: An array or an array-like object of arguments to pass to the function.
Use Case
.apply()
shines in scenarios where you need to invoke a function with an array of arguments, especially useful when dealing with functions that accept variable arguments:
1function introduce(label) {
2 console.log(`${label}: My name is ${this.name}`);
3}
4
5const person = {
6 name: 'Rohan Shakya'
7};
8
9introduce.apply(person, ['Introduction']);
This snippet demonstrates the use of .apply()
to invoke the introduce
function, specifying the this
context as person
and passing an array of additional arguments.
In the Function Prototype
All JavaScript functions inherit .bind()
, .call()
, and .apply()
from Function.prototype
, making them available on every function declared in JavaScript. This inheritance is part of what makes JavaScript's function manipulation capabilities so powerful and flexible.
1function Person(name) {
2 this.name = name;
3}
4
5Person.prototype.greet = function(greeting) {
6 console.log(`${greeting}, ${this.name}!`);
7};
8
9const alice = new Person('Alice');
10const greetAlice = alice.greet.bind(alice);
11greetAlice('Hello'); // Output: Hello, Alice!
12
Conclusion
In conclusion, .bind()
, .call()
, and .apply()
are powerful tools in JavaScript for manipulating function contexts and arguments. Understanding their differences and use cases can significantly enhance your ability to write flexible and reusable code. Whether you need to permanently bind a context, dynamically invoke functions, or pass arguments flexibly, these methods provide the necessary functionality. Incorporating them into your JavaScript toolkit can streamline your code and improve its readability and maintainability.